In modern programming, ensuring code quality and application stability is crucial. One of the best ways to achieve this is through unit and integration testing. Testing is an integral part of the software development process, and its importance grows with the complexity and size of the project.
This article will discuss why testing is so important, the value tests bring, and how they impact code quality and daily work. We will also focus on the practical aspects of testing in the context of the Symfony framework and the PHP Unit tool.
Definitions and types of tests
Unit tests
These tests check individual parts of the code, usually functions or methods, to ensure they work correctly. They are isolated from the rest of the system, allowing quick identification of problems in specific code segments. This makes it easy to locate and fix errors before they are introduced into the larger system.
Integration tests
These tests check whether different modules or components of an application work together correctly. Their purpose is to detect errors that may occur during interactions between different parts of the system. Integration tests cover a broader scope than unit tests as they verify if the system as a whole operates as expected.
Why write tests?
Writing tests is an investment that pays off in the form of higher code quality, easier maintenance, and greater application stability. Here are some key reasons why it is worth writing tests:
Improving code quality
Writing tests forces the developer to think about various scenarios that may occur during application execution. This results in better-thought-out code that is more resistant to errors.
Early error detection
Tests help identify errors at an early stage, reducing the cost of fixing them. Fixing errors detected at later stages of development is usually much more time-consuming and expensive.
Protection against regression
Any change in the code can potentially introduce new errors. Unit and integration tests act as a safety net, detecting unintended side effects of changes. This allows developers to make changes with greater confidence that they won’t break existing functionality.
Facilitating refactoring
Tests provide assurance that after changing the code structure, its functionality remains intact. Refactoring becomes less risky and more efficient, which helps maintain the code in better condition over the long term.
Increasing confidence in the code
Both developers and project stakeholders can have greater confidence in the application, knowing it is well-tested. This increases the assurance that the application will work correctly under various conditions and situations.
Documentation of code
Tests can serve as a form of documentation, showing how the code should work. New team members can quickly understand the expectations for various modules, speeding up the onboarding process and increasing team efficiency.
How tests affect day-to-day work with code
Faster error detection
Automated tests enable quick error detection, allowing for immediate fixes. This lets developers focus on creating new features instead of constantly debugging and fixing errors.
Better team collaboration
In a development team, tests help maintain a consistent coding standard and facilitate project collaboration. Tests provide clear criteria that the code must meet, reducing misunderstandings and conflicts within the team.
Greater confidence during deployment
Automated integration tests allow for quick and safe deployment of changes to production. They help minimize the risk of introducing errors into the production environment, increasing application reliability.
Increased productivity
Although writing tests require additional time, it ultimately saves time that would otherwise be spent debugging and fixing errors. Automated tests can be run repeatedly without additional effort, increasing the efficiency of the code verification process.
Practical aspects of writing tests in Symfony using PHP Unit
Installing PHP Unit
The first step is to install PHP Unit as a development dependency in the Symfony project. This can be done using the composer tool by running the command:
composer require –dev phpunit/phpunit
Creating a unit test
Suppose we have a simple Calculator class in our project that has an add method for adding two numbers. Here is what the class might look like:
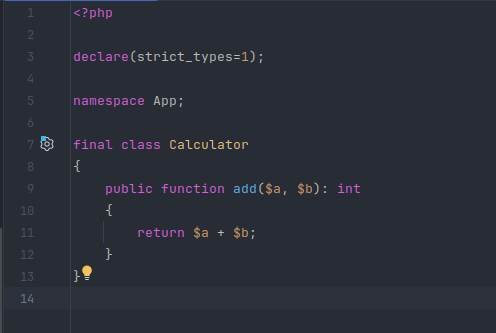
Now, let’s create a unit test for this class:
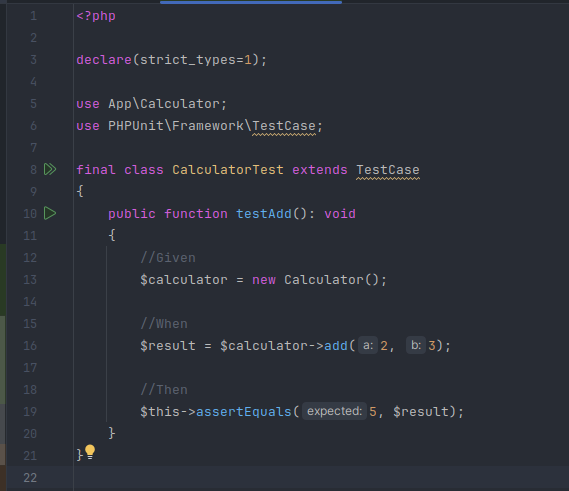
Running the tests
To run the tests, execute the following command in the terminal:
./vendor/bin/phpunit
If the tests pass successfully, you will receive a confirmation message. Otherwise, you will be informed of the errors that need to be fixed.
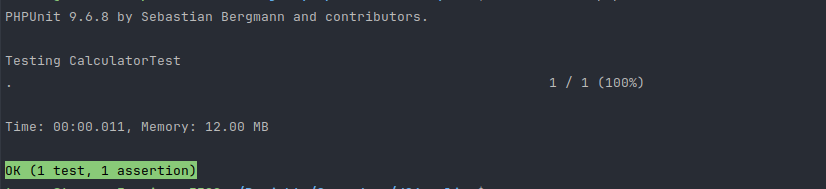
Integration tests
Integration tests are more complex because they involve interactions between different components of the system. Suppose we have a controller in Symfony that uses the Calculator class.
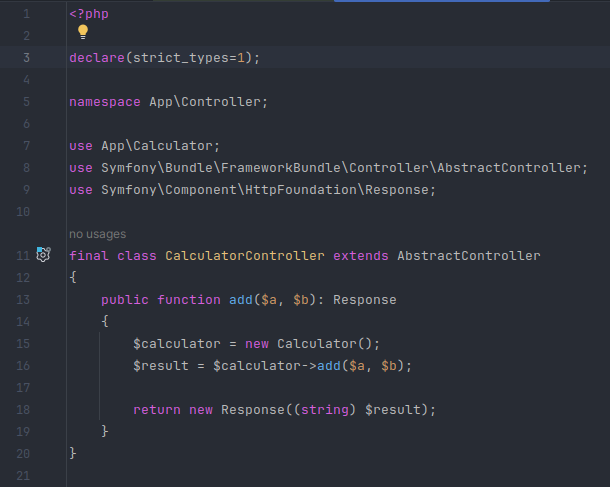
Now, let’s create an integration test to ensure our controller works correctly:
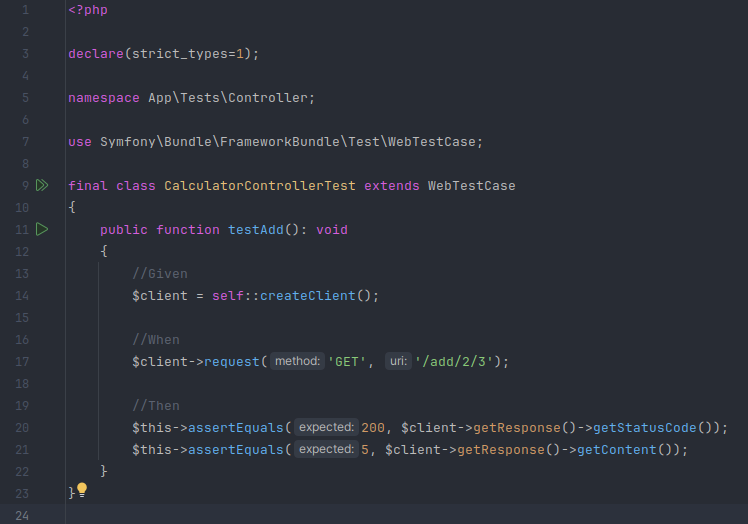
Similar to unit tests, run the integration tests with the command:
./vendor/bin/phpunit
Conclusion
Unit and integration testing are an essential part of the modern software development process. Tests improve code quality, protect against regression, facilitate refactoring, and increase confidence in the application. In a developer’s daily work, tests are a tool that speeds up error detection and allows for safe changes, leading to more stable and efficient applications. In the context of the Symfony framework and the PHP Unit tool, writing tests is simple and effective, enabling the easy adoption of good testing practices in any project.